In the
previous example, you can see the purpose of a structured data
object. In C, you can create a data type to represent the
previous variables, however, it must be declared. How? It is
explained in the next section.

This can be
illustrated through a list of steps. Considering the database
example above, the steps included are to:
1
Determine what fields we wish to have in our record.
2
Determine the data type for each variable that will be
used in each of the fields.
3
Determine the
amount of bytes to be assigned to each of the fields.
4
Declare the record in C according to the fields and
variables selected in the previous steps.
The following
segments of a C program illustrate these steps in executable
code:
struct
Customer Info
{
char
Name [31];
char SSN [10];
char DOB [8];
char Address [41];
int
Age [2]; };
int
main()
{ struct
Custmer Info active =
{"Favre,
Brett",12345,"CIS",3.27,67};
struct
student alumni =
{"White, Reggie", 23456, "Pain", 1.34, 89};
printf("Name:
%13s %14s\n",active.student_name,
alumni.student_name);
printf("ID:
%13d %14d\n",active.student_id,
alumni.student_id);
printf("Major:
%13s %14s\n",active.major,
alumni.major);
printf("gpa:
%13.3f %14.3f\n",active.gpa,
alumni.gpa);
printf("Hours:
%13d %14d\n",active.total_hrs,
alumni.total_hrs);
return 0;
}
Why are Name
and Address given an odd number of bytes? Is this the desire of
the programmer or is there a reason for this choice?
The truth is
that without the extra byte given to “Name” and “Address” we
would need to keep track of the string size. However, by
previously adding a byte to allow a null character, keeping
track of a string size is not necessary.
The way a
struct is declared in C can be
compared to the way it is declared in other languages since
there are some similarities. Take for instance, the following
structures in their respective languages:
In COBOL
01 Customer
Info
02 Name PIC
X(40).
02 SSN
PIC 9(9).
02 DOB
PIC 9(6).
02 Address PIC
X(35).
02 Occupation PIC
X(30).
02 Age
PIC 9(3).
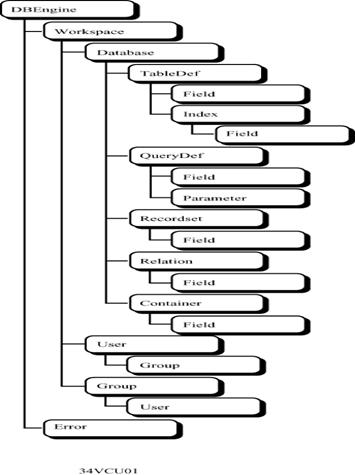
In PASCAL
Type
Customer Info = record
Name : array [1..40]
char
SSN :
interger;
DOB : integer;
Adress
:array[1..35] of char;
Occupation : array
[1..30];
Age : integer;
end;
In SQL
create
table student
(
student_name
char(30) not null,
student_id integer
not null,
major
char(10) default ‘CIS’,
gpa
decimal(4,2),
total_hours
smallint,
primary
key (student_id),
check
(gpa >
0) )
In JAVA
{
int
i = 3;
public static void main (String [] args)
{
CODemo2 obj1 = new CODemo2 ();
System.out.println ("obj1.i =
" + obj1.i);
obj1.printHello ();
CODemo2 obj2 = new CODemo2 ();
obj1.i = 5;
System.out.println ("obj1.i =
" + obj1.i);
obj1.printHello ();
System.out.println ("obj2.i =
" + obj2.i);
obj2.printHello ();
}
void printHello ()
{
System.out.println ("Hello!
i = " + i
+ "\n");
}
}
Learning the
basics of structured data objects is important in any language.
As seen in the previous examples of code, how you declare them
depends on the syntax (or rules) of the language.

1. Why is
there an extra byte allocated for Name
and Address if they should
consist of 30 and 40 correspondingly?
The reason for
this, is because we are storing these
fields as the data type character array, which means that we
need to reserve one extra byte for the
null character. This is necessary,
otherwise we would have to keep track of the length of each of
the strings Name and Address.
2. Suppose
I have a Struct named “struct
thierteenless1” and it has the following elements:
char
student_lastname[31]
char
student_firstname[11 ]
int
age
int
accumulated_hours
float
gpa
How many
total bytes of storage are used
in RAM?
a)
10 contiguous bytes of storage.
b)
10 non-contiguous bytes of storage.
c)
50 contiguous bytes of storage.
d)
50 non-contiguous bytes of storage.
ANSWER: C
3. What is
the primary difference between a
Struct and an
Array?
a)
There is no difference they are the same.
b)
A Struct can only have one data
type.
c)
A Struct can have several data
types.
d)
A
array can have does not need contiguous space.
ANSWER: C

http://www.le.ac.uk/cc/tutorials/c/ccccstrc.html#dt
http://msdn.microsoft.com/library/default.asp?url=/library/en-us/csref/html/vcwlkStructsTutorial.asp
http://www.cprogramming.com/tutorial/lesson7.html
http://www.problemsolvingcpp.jbpub.com/Dale_Chapter11.pdf#search='c%20structured%20data%20objects