What is RAM?
By definition Random
Access Memory is the place in a computer where the
operating system, application programs, and data in current use
are kept so that they can be quickly reached by the computer's
processor. RAM is much faster to read from and write to than the
other kinds of storage in a computer, the hard disk, floppy
disk, and CD-ROM. However, all the data stored in RAM is only
stored while the computer is running, after you shut
it off all data is erased.
First, let us remember
that characters require 1 byte of storage in RAM or 8 bits. On
the other hand, storing integers (the data type int)
require 2 CONTIGUOUS bytes (16 bits) of storage in RAM. Now
lets consider the following example:
C
declaration:
int a, b = 'W', c= 1543;
652 |
653 |
654 |
655 |
656 |
657 |
658 |
avail. |
avail. |
avail. |
in use |
in use |
avail. |
avail. |
658 |
659 |
660 |
661 |
662 |
663 |
664 |
in use |
in use |
in use |
in use |
avail. |
avail. |
avail. |
We have declared the
variables a, b, and c as integers (data type int),
meaning that they require 2-bytes (16 bits) of storage each. We
are requesting a total of 6-bytes (48 bits; 16-bits per
variable) be set aside in RAM at locations a, b, and c.
Let us assume that
locations 652, 653, 654, 657, 658, 662, 653 are available (i.e.
655, 656, 658-661 are being used). In this case, variable a
will be assigned to address 652 (and 653), variable b
will be assigned to address 657(and 658) and variable c
will be assigned to 662 (and 663), and initialized with the
value 1,543.
The reason numbers are
skipped is that integers require 2 contiguous bytes of memory.
Because some of the addresses are not available, they are
skipped and go on to the next available storage.
Locations 652 and 653 already have a value
associated with them. Even though we have not yet stored
anything there, what was previously stored there is still there.
Contrary
to other programming languages C and C++ do not clear the
contents of an address when a variable is assigned to a
particular address. Other programs, like Cobol, when allocating
a variable to an address, get rid of whatever is stored at that
particular address. C and C++ keep whatever value is stored in
that address until we actually set a value to the variable.
Keep in
mind that c programming language allows for additional integer
data types. Such as:
unsigned integers.
This allows the storage of all values from 0 to 65,535 (216
- 1), 16-bits(2-bytes)
longs. This allows
the storage of all integers from (-2,147,483,648 to
2,147,483,647 (-231 to 231
-1), 32-bits(4-bytes)
unsigned longs. This
allows for the storage of all value from 0 to 4,294,967,296 (232
-1), 32-bits(4-bytes)
*Unsigned
integers are stored exactly the same as integers except that we
never have to worry about complimenting.
Let's not forget that integers are really
stored on 32-bits.
One more
time for reviewing sake, if we assume that an integer is stored
in a particular location in RAM and we go and look at that
address we will find 1 byte or 8 bits. The contents of that
particular address are determined by whether if we have
initialized the variable or not. If we did initialize it the
contents will be whatever value we assigned to the variable; if,
on the contrary, we did not initialize the variable we will get
whatever value was stored at the location also called garbage.
Now let’s test your understanding with the following questions: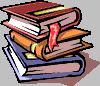
If we see
the following statement unsigned int how is the computer
going to evaluate the contents of that location?
Answer:
The statement unsigned int will result in the computer
evaluating all 8 bits to come out with the numeric value stored
in the location.
Why do we
get such strange output when printing out the contents of a
declared ? And why does this happen?
Answer:
Because by declaring a variable we are only requesting a
location in RAM, we are not initializing it. Therefore, when
printing out the contents of that variable, C++ will give us
whatever character was stored there before. Remember that C and
C++ do not clear the contents of the location in RAM when
allocating an address.
How many
contiguous bytes of storage would a long require?
A- 2 B- 4 C-6
Answer:
B. A long would require 4 contiguous bytes (32-bits) or storage.
Can we
print out an integer as a character?
Answer:
Yes, if a variable contains a certain value, we look at the
rightmost 8-bits, determine the numeric value stored there, and
then print out the corresponding ASCII character.
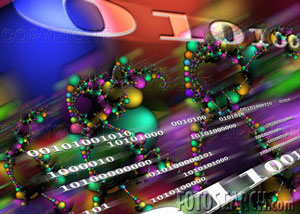
Here are a
few References:
http://www.quepublishing.com/articles/article.asp?p=330332&rl=1
http://lib.daemon.am/Books/C/ch03/ch03.htm
Programming in C and C++