-
The
variable x is stored in address 1245 & 1246.
-
The
variable y is stored in address 1250 & 1251.
-
The
variable z is stored in address 1254 & 1255.
BUT WHY IS THERE A VALUE IN ADDRESSES 1254 & 1255 IF WE HAVE NOT
STORED ANYTHING IN THERE????
Well, very
simple that is because in c data is stored temporarily but when it
is used the locations are not resetted to zero instead they keep the
information that was previously stored until you allocate new values
into the locations. That means that if we were to print the
contents of this two locations it would print whatever information
was stored there before.
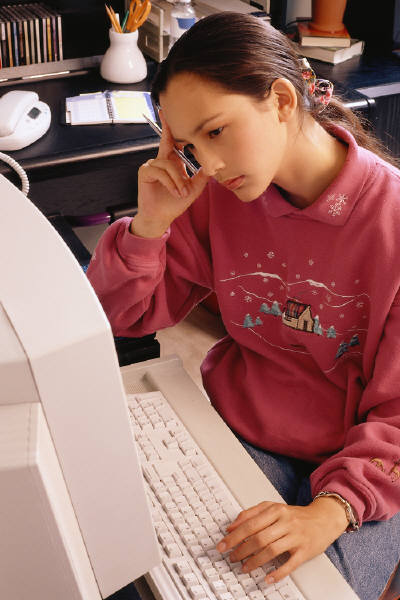
You may have
notice that variable x contains a character which only needs 7 bits
of storage. But, how can it be stored as an integer???
The only difference
is that integers require 16 bits of storage so in order to store a
character as an integer we would need to add zeros before the fist
number one until we complete the 16 bits. For example in this case
the character J is stored as 00000000 01001010. In the same way we
can print out an integer as a character in doing so we would look at
the rightmost 8 bits, determine the numeric value stored there and
the print out the corresponding ASCII character.
What would
happen if we assign a value like 55,456 to an integer
variable???????????
55, 456 is an
illegal value because remember that integers only take from the
range of values -32,768 to 32,767. However, c is a program that
even when given an illegal value it will perform an operation. In
this situation the program will do the following:
1. First it
convert the decimal value into binary:
55,456 = 1101100010100000
2. Then it
will interpret that number. First, since the first number is 1 the
number appears to be negative.
3. Then to
evaluate we take the two's complemented number:
101100010100000
010011101011111
+
1
010011101100000
4. Then we
determine the value of 10011101100000 to be -10,080.
If we really need
to store integer values such as the one used in our previous example
or even greater than that c programming language allows for the use
of additional integer data type:
UNSIGNED
INTEGERS; which allow to store values form 0 to 65,535.
LONGS; which
allow to store values from -2,147,483,648 to 2,147,483,647.
UNSIGNED LONGS;
which allow to store values from 0 to 4,294,967,296.
These additional
types of integers are stored the same as regular integers, with the
only difference that with unsigned integers and longs you don't have
to worry about complementing.
USEFUL
INFORMATION
Variable Types.
Type |
Size |
Values |
unsigned
short int |
2 bytes |
0 to 65,535 |
short int |
2 bytes |
-32,768 to
32,767 |
unsigned
long int |
4 bytes |
0 to
4,294,967,295 |
long int |
4 bytes |
-2,147,483,648
to 2,147,483,647 |
int (16
bit) |
2 bytes |
-32,768 to
32,767 |
int (32
bit) |
4 bytes |
-2,147,483,648
to 2,147,483,647 |
unsigned
int (16 bit) |
2 bytes |
0 to 65,535 |
unsigned
int (32 bit) |
2 bytes |
0 to
4,294,967,295 |
char |
1 byte |
256 character
values |
float |
4 bytes |
1.2e-38 to
3.4e38 |
double |
8 bytes |
2.2e-308 to
1.8e308 |
******TEST
YOUR KNOWLEDGE******
(Answers at
bottom of the page)
Multiple choice questions
1.
If locations 2653, 2654, 2655 and 2656 are the only locations we can
use, and location 2654 contains the numeric
value
100. If I requested unsigned int a='A', What would happen?
a)
use location 2653 and 2655 because integers need two bytes, location
2654 is being used.
b)
use 2653, clear location 2654 and use them both to store the
integer.
c) It cannot be done because integers need two contiguous
bytes and 'A' is a character which means that it only
needs one by of storage.
d)
use 2655 and 2656 and store the binary
number 0000 0000
0100 0001
2. Regardless whether signed or unsigned, the data type int
requires ___________ of storage per variable.
A) Two bytes
B) Three bytes
C) Sixteen bits
D) A and C
E) None of the above
3 If in the binary values 00011010 and 00111111 are stored in
the addresses 2567 and 2568 correspondingly, what
decimal value is stored at address 2568 if the data type
is an integer?
a) 63 because this is the ASCII decimal value
associated with the binary value 00111111.
b) -65
c) 6719 because integers require 2 bytes of
contiguous memory, so both addresses 2567 and 2568 would have to
be evaluated in order to determine the answer.
d) None of the above, we do not have enough
information to determine the answer.
Short answer questions
1. What is the difference between storing
a character versus an integer in RAM?
2. Can an integer be stored in any
available space in RAM?
3. How is a variable address referred to
in RAM?
4. What happens if I assign a
negative number to an unsigned variable? Consider the following line
of code: