How
do we know where a piece of datum is stored in RAM?
Although the answer to this question is that we
don't know, because the address allocations in RAM
are made at RUN-TIME and are based on available locations, we can
still determine the address location of a variable using some c/c++
commands.
For example, lets declare a variable char x,
and use the command %lu to find its location in RAM:
#include <stdio.h>
void main()
{
char x;
printf ("the RAM location of variable x is %lu
understand?\n",x);
}
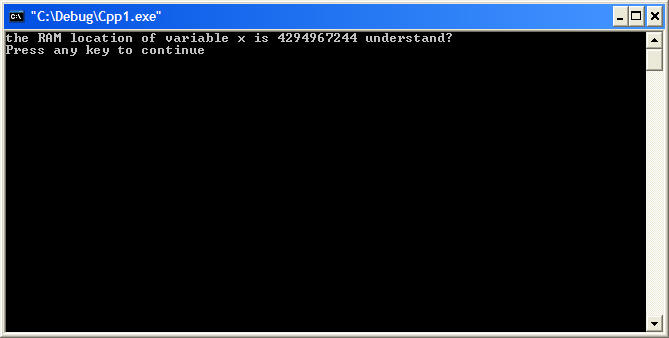
In the example above, the variable was declared
as of type "char" which as we know only requires 1 byte of storage
(in this case was the location 4294967244), if instead we use a
variable of type "short", the variable will require 2 contiguous
bytes, so forth for the different types of variables.
In some cases, in order to find some difficult
locations we are forced to calculate the location utilizing some
formulas.
One good example of calculate a location of a variable in ram is
when using an Array.
For example, lets first create an array type
short of 10 elements, and use the command %lu to find its base
address in RAM:
#include <stdio.h>
void main()
{
short arrayX [10] = {1,2,3,4,5,6,7,8,9,10};
printf ("the RAM base address of arrayX is %lu understand?\n",arrayX);
}
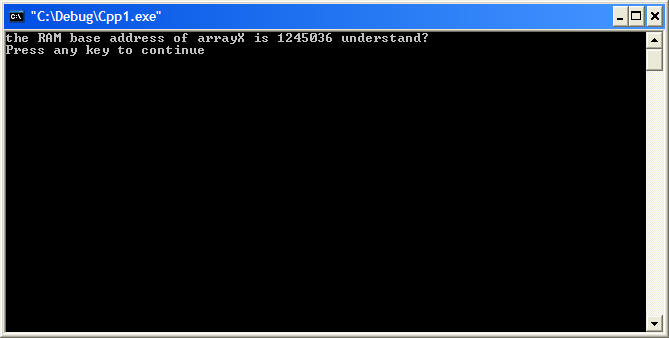
In the example above, the array was declared as
of type "short" which as we know requires 2 bytes of contiguous
storage (in this case the first location or base address was
1245036), therefore we know that the first element of the arrays
will occupy the locations 1245036 and 1245037, the second element of
the array will occupy the locations 1245038 and 1245039, and so
forth for all the elements of the array. As mentioned before, there
is a better way to determine the location of an element in an array
using a formula.
for example, in order to determine the location
of the 2nd element (notice that the first element is 0) in this
array will apply the formula:
Base Address + ( Offset * #bytes per element) = location
1245036 + ( 3 * 2 ) = 1245042
We can check this by modifying the
program
#include <stdio.h>
void main()
{
short arrayX [10] = {1,2,3,4,5,6,7,8,9,10};
printf ("the location of the 3rd element of arrayX is %lu
understand?\n",&arrayX[2]);
}
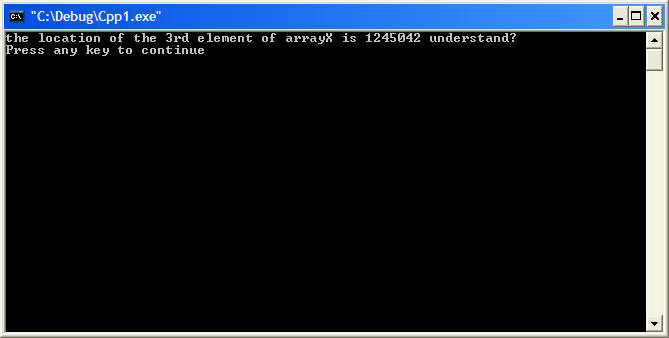
what is an Array? A data
structure containing a fixed number of contiguous storage elements
all of the same type
What is Random Access Memory?
Random Access Memory is used to temporarily store information that
the computer is working with. It is the component in the computer
where most programs are loaded. These programs in RAM perform their
functions and operate to give the user the required results.
Most RAM memory is stored in chips (integrated circuits ICs) that
are found on the motherboard of a computer. Memory chips are
normally only available as part of a card called a module. Below are
examples of a SIMM (single in-line memory module), a DIMM (dual
in-line memory module), and a SODIMM (small outline dual in-line
memory module) from left to right respectively.
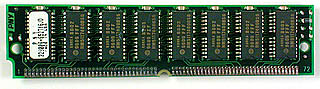
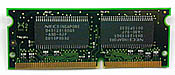
How does RAM store data?
RAM consists of many capacitors
and transistors. A capacitor and a transistor are paired together to
make a memory cell. The capacitor represents one "bit" of data, the
transistor is able to change the state of the capacitor to either a
0 or a 1. the Zero's and ones when read in a sequence represent the
code which the computer understands. This is called binary data
because there are only two states that the capacitor can be in.
When we reserve bits of storage for a given datum, for example in
the following C Declarations:
Remember, characters require 8-bits of storage (1-byte), shorts
require 16-bits of storage (2-bytes), and integers/longs require
32-bits of storage (4-bytes).
1.
we know that
we are reserving 7-bytes of storage ? 1-byte for a, 2
contiguous bytes for b, and 4 contiguous bytes for c
2.
we are
associating the locations with variable names (Location a,b,c)
3.
we are
initializing location a with the character ?c? (ASCII = 99,
011000112), location b with the value 50
(00000000-001100102), and location c with the
value 5487 (00000000-00000000-00010101-011011112)
So the question is where in RAM will we find location a, b, and c?
Address allocations are made at RUN-TIME and are based on
available locations. At this point we don?t know where a, b,
and c are stored. The table below is an illustration of where
the above variables may be stored in RAM.
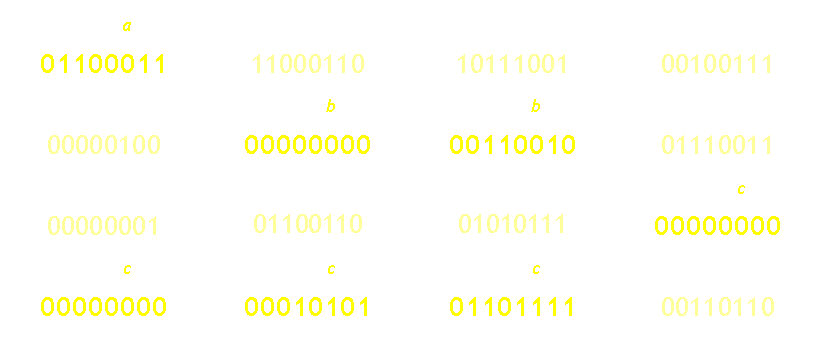
For more detailed information on RAM allocation (references I used),
here are a few sites to look at:
http://en.wikipedia.org/wiki/Random_access_memory
http://en.wikipedia.org/wiki/Datum
http://www.howstuffworks.com/ram1.htm
http://www.ece.utexas.edu/~valvano/embed/chap3/chap3.htm
http://www.allsands.com/Computers/whatisramrand_wde_gn.htm
http://www.howstuffworks.com/ram1.htm
Practice multiple choice and short answer questions:
1. How many bytes/bits of storage would a data type float
require?
a)
2
contiguous bytes
b)
4
contiguous bytes
c)
16
bits
d)
32
bits
e)
both
a and c
f)
both
b and d
2.
How many contiguous bytes/bits of storage would a data type
double require?
a)
64
bits
b)
16
bytes
c)
32
bits
d)
8
bytes
e)
a
and d
f)
none
of the above
3.
How many contiguous bytes/bits of storage would a data type
long double require?
a)
32
bytes
b)
32
bits
c)
128
bytes
d)
128
bits
e)
a
and d
f)
none
of the above
4. Which
of the following statements is true about RAM?
a) to hold the program code and data during execution
b) its accesses to different memory locations are almost always
completed at about the same speed.
c) it is a random access memory
d) A and C
e) All of the above
Answers:
1.
f.
2.
e.
3.
d.
4. e.