Bits/Bytes and ASCII Part 1
Type-in, Compile, and
Run the Program (5 point)
For this part of
the project, all you have to do is type in, compile
and run the program listed below (there are three trivial syntactical errors; I
tell you where they are).
You will notice that the program is loaded
with comments. This is to help you understand what the program does. You do not
have to include all of the comments (unless you want to, or you want to add
some) EXCEPT that you MUST add the first 5 comments (appearing before the
#include statement). These lines MUST appear in every program you
write and turn in.
On additional caveat (although I have said it
before, and will say it again): You could cut and paste this code, and then
modify it accordingly. What will you learn if you do?? Will it help you on
the quizzes?? In a job?? Are you really so desperate for time that you can't
afford the 5 minutes extra it will take you to type it in?
Type in, compile and run the following c program.
// Program Name: <The name you decide upon
for the program>
// Author: <Enter your name here>
// Date Written: <Enter the date you first started
the program>
// Last Modified: <Enter the date you last modified
the program>
// Intent: Set up an initial Menu
#include <stdio.h> // Include
the Standard C Input-Output (IO) Headers File
int main(void)
// MAIN is a
function name which returns an integer
{
// This is similar to a BEGIN statement
char ch,ch1;
// ch and ch1 are
the
variables where we will store ASCII characters
// BUT
they are really signed numeric bytes (on 8-bits)
unsignd char ch2; // ch2 is also a
location holding an ASCII character
** There is an error here
// BUT it is an unsigned numeric byte
ch = 'T';
// Store the ASCII Character T
(numeric value 84) at location ch
// Let's print out the contents of location ch as a
character, decimal, octal and hex
printf("The values of character %c are %d decimal, %o octal and %x
Hexadecimal\n",ch,ch,ch,ch);
// Let's change the contents of location/variable ch so that
it contains the value 38
ch = 38;
// Again, print out the contents of ch as a character, decimal, octal and hex
*** There is an error
printf("The values of character %c are %d decimal, %o octal and %x
Hexadecimal\n,ch,ch,ch,ch);
// This time we are going to change the contents of ch by
storing an Octal value in it
// Of course, we know that we really can't store an Octal value (ALL values are
stored in Binary)
// The compiler will change our Octal entry to Binary
// We indicate that it is an Octal value by putting a 0 (Zero) in front of the
number
ch = 0135;
// Let's get the same output we have been getting *** There
is an error here
printf(The values of character %c are %d decimal, %o octal and %x
Hexadecimal\n",ch,ch,ch,ch);
// This time we are going to change the contents of ch by
storing a Hexadecimal value in it
// Again, we know that we really can't store an Hex value (ALL values are stored
in Binary)
// The compiler will change our Hex entry to Binary
// We indicate that it is an Hex value by putting a 0X (Zero followed by 'X') in
front of the number
ch = 0X6b;
// Let's print it out again
printf("The values of character %c are %d decimal, %o octal and %x
Hexadecimal\n",ch,ch,ch,ch);
// Let's add two characters together and see what happens
ch = '3';
// Assign the Character 3
ch1 = 52;
// Assign the Character 4
ch = ch + ch1; // Add the two together - What
is the outcome?
// Let's print it and find out
printf("The values of character %c are %d decimal, %o octal and %x
Hexadecimal\n",ch,ch,ch,ch);
//
Now, let's try and store an
'Illegal' value in location ch
// I say illegal because we know that signed bytes can only take on the values:
// -27 through +27 - 1, or -128 though +127
// What happens if we try and store a number outside of that range?
ch = 211;
// Let's print, using a slightly different format, and find out
printf("The outcome is %c, decimal %d\n",ch,ch);
//
Now, if we were to store the
value 211 at location ch2 (an unsigned character) it should be OK
// since an unsigned character has the range 0 through 28 - 1 (0 through 255)
// Let's try it
ch2 = 211;
// Let's print it out again (What ASCII Character corresponds
to the decimal value 211??)
printf("The outcome is %c, decimal %d\n",ch2,ch2);
// OK - we're done.
// But remember, we declared our main function to be an integer function
// Although we could get by without returning an integer value (we would get a
warning message)
// For the sake of correctness, we might as well return a 'dummy' value
return(0);
// Now let's just end the main function
// How?? Remember, we stated the function with a {,
so we need to end it with a matching }
}
If you entered the code correctly (and fixed the
intentional errors I told you about), you should see an output screen that looks
like:
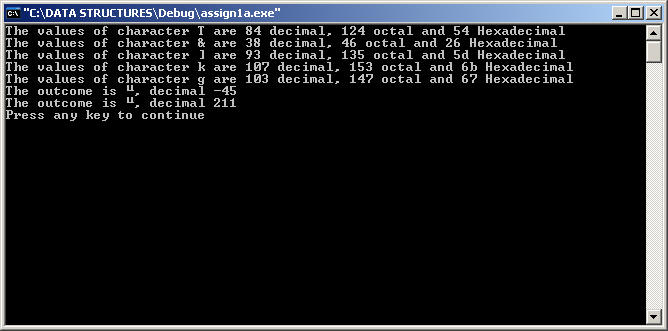
To run the executable code, click here.
CAVEAT: Depending on your browser, the sample code may
flash by.
Make sure to submit your
programs via the Submission Page by the
Date Due (See Course Schedule)

This page was last updated on
07/06/05