Bits/Bytes and ASCII Part 2
Modify the Program so
it will print your name (10 point)
NOTE: Do NOT merely go
back and modify Part 1 of the assignment. Either open a new file and cut and
paste the commands from the previous assignment, OR open the previous
assignment, save it as a different file, and then modify it. In other words,
each part should be a different file. These instructions hold
for ALL of the programming assignments given.
As the heading states, all you have to do is
modify the program so it will write your name. There is a catch, however (isn't
there always?):
You MUST use the following pattern
in entering your name:
letters: 1, 5, 9, 13,
. Enter the input as characters
letters: 2, 6, 10, 14,
. Enter the input as integers
letters: 3, 7, 11, 15,
. Enter the input as octal numbers
letters: 4, 8, 12, 16,
. Enter the input as hexadecimal numbers
Where do you enter the Code??
If you will remember, the last command we used in the
previous part was:
return(0);
}
Enter your new commands Just before the
return statement.
For example, for my name (Peeter Kirs), the code I would
use is (again, all of the comments I have included are only
to help you better understand what is going on):
ch = P;
// I store the ASCII code for 'P' in location ch (letter 1)
printf ("%c",ch);
// I Print out the ASCII Code stored in location ch ('P')
ch = 101;
//
I store the numeric value 101 (ASCII 'e') in location ch (letter 2)
printf ("%c",ch);
// I
Print out the ASCII Code stored in location ch ('e')
ch = 0145; //
I store the OCTAL value 145 (Decimal 101; ASCII 'e') in location ch (letter 3)
printf ("%c",ch);
// I Print out the ASCII Code stored in location ch ('e')
ch = 0X74; //
I store the HEX value 74 (Decimal 117; ASCII 't') in location ch (letter 4)
printf ("%c",ch);
// I Print out the ASCII Code stored in location ch ('t')
// Before proceeding, notice that I entered: The 1st piece of datum as a
character,
// The second as a decimal value, the third as an Octal value, and the 4th as a
Hex value
// I now repeat that pattern for the rest of my name
ch = e;
// I store the ASCII code for 'e' in variable ch (letter 5; same format as
letter 1)
printf ("%c",ch);
// I Print out the ASCII Code stored in variable ch ('e')
ch = 114;
// I store the numeric value 114 (ASCII 'r') in variable ch (letter 6; same as
letter 2)
printf ("%c",ch);
// I Print out the ASCII Code stored in location ch ('r')
ch = 040;
// NOTE: This is the space which
separates My first & last name
printf ("%c",ch);
ch = 0X4B
// I store the Hex value 4B (Decimal 75; ASCII 'K') in location ch (letter 7)
*** There is an error here
printf ("%c",ch);
// I Print out the ASCII Code stored in location ch ('K')
ch = i;
// I store the ASCII code for 'i' in variable ch (letter 8; same format as
letter 1)
printf ("%c",ch);
// I Print out the ASCII Code stored in location ch ('i')
ch == 0164;
// I store the OCTAL value 164 (Decimal 114; ASCII 'r') in location ch (letter
9) *** There is an error
printf ("%c"); // I Print
out the ASCII Code stored in location ch ('r') *** There is an error here
ch = 0X73; //
I store the HEX value 73 (Decimal 116; ASCII 's') in location ch (letter 10)
printf ("%c",ch); // I Print
out the ASCII Code stored in location ch ('s')
printf ("\n");
//
Print-out a carriage return
That's it. If you have a long name, you will need more
lines of code. If you have a short name, fewer lines of code.
The output would appear as:
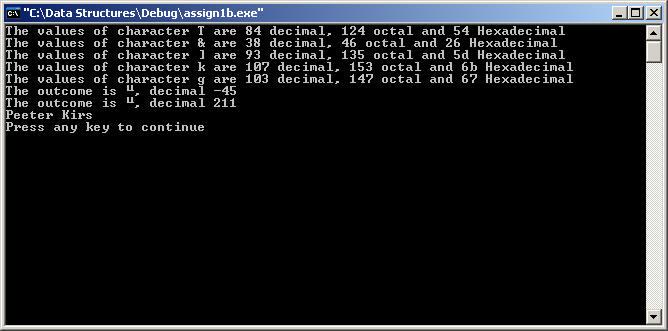
To run the executable code, click here.
CAVEAT: Depending on your browser, the sample code may
flash by.
Make sure to submit your
answers via the Submission Page by the
Date Due (See Course Schedule)

This page was last updated on
07/06/05