Functions
The intent of this program is to learn about how functions
work. We are going to take your previous program and clean it up using
functions. There is only one part to this assignment.
I am going to give you
my main function, and all of the include and function prototypes I used:
#include <iostream.h>
void displaymenu();
void checkchoice(char choice);
int getaninteger(short number)
float getafloat(short number);
void addintegers(int int1, int int2);
void subtractintegers(int int1, int2);
void multiplyintegers(int int1, int int2);
void divideintegers(int int1, int int2);
void getquotient(int int1, int int2)
void getremainder(int int1, int int2);
void multiply(float float1, float2);
void divide(float float1, float float2);
void main()
{
char menuchoice;
int intvalue1; intvalue2;
float fvalue1, fvalue2
// Present the Main menu
displaymenu();
// get the user selection
cin >> menuchoice;
// Check the user selection
checkchoice(menuchoice);
// Get user entered integers
if ((menuchoice >= '1) && (menuchoice <= '6'))
{
intvalue1 = getaninteger(1);
intvalue2 = getaninteger(2);
}
// Get user entered reals
if ((menuchoice == '7') || (menuchoice = 8));
{
fvalue1 = getafloat(1);
fvalue2 = getafloat(2);
}
// Perform the operation requested
switch (menuchoice)
{
case '1' : addintegers(intvalue1,
intvalue2); break;
case '2' :
subtractintegers(intvalue1, intvalue2); break;
case '3' :
multiplyintegers(intvalue1, intvalue2); break;
case '4' : divideintegers(intvalue1,
intvalue2); break;
case '5' : getquotient(intvalue1,
intvalue2);
case '6' : getremainder(intvalue1,
intvalue2); break;
case '7' : multiply(fvalue1,
fvalue2); break;
case '8' : divide(fvalue1, fvalue2);
break;
}
}
Are there errors? A few, but they are easily
corrected.
Let's take a look at a few of the functions.
void displaymenu()
Remember the basics of functions:
- They must have a unique name (in this case
displaymenu)
- They May require arguments or parameters (or may not,
as in this case)
- They may return a value (or may not, as in this case)
What does this function do? It presents the menu to the
user. It is almost trivial. In fact, I will even give it to you:
void displaymenu()
{
cout << "Enter your choice:\n\n";
cout << " 1. Add two integers\n";
cout << " 2. Subtract an integer from another integer\n";
cout << " 3. Multiply two integers\n";
cout << " 4. Divide two integers\n";
cout << " 5. Find the Quotient of two integers\n";
cout << " 6. Find the Remainder of two integers\n";
cout << " 7. Multiply two Real Numbers\n";
cout << " 8. Divide two Real Numbers\n";
cout << "? ";
}
The only other thing you should keep in mind is that all
functions come after the main function. Make sure you add the
function after the end of the main function.
int getaninteger(short number)
Again, this function:
- Has a unique name (in this case getaninteger)
- Passes an argument or parameter (in this case, a short
integer)
- Returns an integer value
How does it work? Let's look at how it is used in the
code:
if ((menuchoice >= '1) && (menuchoice
<= '6'))
{
intvalue1 = getaninteger(1);
intvalue2 = getaninteger(2);
}
As you will remember, if the user chose any of the menu
choices '1' through '6', that meant they wanted to perform an operation using an
integer. That meant that the first thing we had to do was get the two integers
they wanted to work with.
What are we passing the function? In this case the
number of the integer (either 1 or 2). This might make more sense if we look at
the output:
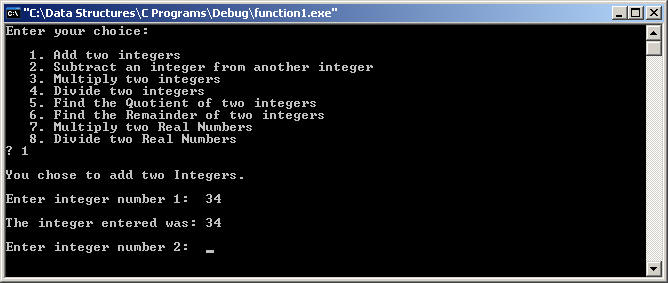
The first time we call the function (intvalue1
= getaninteger(1);), we pass it the value 1. Hence the output:
Enter integer number 1:
The second time we call the function (intvalue2
= getaninteger(2);), we pass it the value 2. Hence the output:
Enter integer number 2:
What does it return? An integer value.
That is what we said it would return when we declared it as:
int getaninteger(short
number)
What does it do with it? The first
time it is called it places the integer in location intvalue1 (intvalue1
= getaninteger(1);); the second time it is called, it places it in intvalue2
(intvalue2 = getaninteger(2);)
Once again, I'll even give you the code for
the function:
int getaninteger(short number)
{
int aninteger;
cout << "\nEnter integer number " << number <<": ";
cin >> aninteger;
cout << "\nThe integer entered was: " << aninteger << endl;
return(aninteger);
}
Pretty straight-forward. As for the other functions, well, I think you can
figure them out on your own. For the most part, it is simply a situation of
taking them out of the main function, and setting them up as functions of their
own. We can, however, look at some of the parameters passed and values returned
for each of the functions:
Function Name |
Description |
Values passed |
Values Returned |
addintegers
|
Add two integers together
|
two integers
(intvalue1 and intvalue2;
received as int1 and int2) |
nothing (void)
Prints out the result of:
int1 + int2 |
checkchoice
|
Check to see if a valid
choice was made
|
one character
(menuchoice;
received as choice) |
nothing (void)
|
displaymenu |
Display the User Menu |
None |
nothing (void) |
divide
|
Divide two real numbers
|
two floats
(fvalue1 and fvalue2;
received as float1 and float2) |
nothing (void)
Prints out the result of:
float1/float2 |
divideintegers
|
Divide two integers yielding a real
result
|
two integers
(intvalue1 and intvalue2;
received as int1 and int2) |
nothing (void)
Prints out the result of:
(float) int1 / (float) int2 |
getafloat
|
Gets a real number from the user
|
one short integer
(used to print integer number;
received as number) |
one real number
received as either:
fvalue1 or fvalue2 |
getaninteger
|
Gets an integer from the user
|
one short integer
(used to print integer number;
received as number) |
one integer
received as either:
intvalue1 or intvalue2 |
getquotient
|
Gets the quotient of 2 integers
|
two integers
(intvalue1 and intvalue2;
received as int1 and int2) |
nothing (void)
Prints out the result of:
int1 / int2 |
getremainder
|
Gets the remainder of 2 integers
|
two integers
(intvalue1 and intvalue2;
received as int1 and int2) |
nothing (void)
Prints out the result of:
int1 % int2 |
multiply
|
Gets the product of 2 real numbers
|
two floats
(fvalue1 and fvalue2;
received as float1 and float2) |
nothing (void)
Prints out the result of:
float1 * float2 |
multiplyintegers
|
Gets the product of 2 integers
|
two integers
(intvalue1 and intvalue2;
received as int1 and int2) |
nothing (void)
Prints out the result of:
int1 * int2 |
subtractintegers
|
Gets the result of one integer subtracted
from another
|
two integers
(intvalue1 and intvalue2;
received as int1 and int2) |
nothing (void)
Prints out the result of:
int1 - int2 |