Numeric/Character Arrays Part 2
Let's write the code
necessary to carry out the menu options listed in part 1:
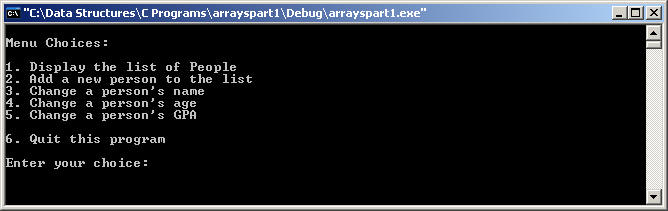
Choice 1: Display
the list of people.
This is pretty simple.
We know how many people there are (we stored this value in variable/location
numberpeople). Now all we have to do is print out the list using a
for loop. I added the variable offset (as a short integer) into my
list of variable declarations for this purpose. So the basic command is
simply:
for (offset = ??;
offset < ??; ??)
I think you can figure
out what is missing. Here is how my output appears:
You'll notice that
after I finished listing the people, I prompted the user to return to the
main menu, and we did.
You will also notice
that my output is formatted. Since we didn't spend much time in formatting,
I will give you the print string:
printf("%-20s %3d
%5.3f\n,personname[offset], personage[offset], persongpa[i])
There are easily fixed
errors in the code above.
Choice 2: Add a new
person to the list
Again, this is pretty
simple. We already know that there numberpeople on the list. All we
have to do stored at get the user's input and store them at
index/offset [numberpeople]. Then we have to increment the value of
numberpeople.
That might initially
sound strange (storing the values at offset numberpeople and THEN
incrementing the value. But think about it. Right now, the value of
numberpeople is 5 (five), but they on stored on offsets [0] ... [4]. We
want to store our new values at offset [5] (the present value stored at
location numberpeople), and then increment the value stored at
numberpeople to 6 (the number of people NOW on our list).
Here is how my program
did it:
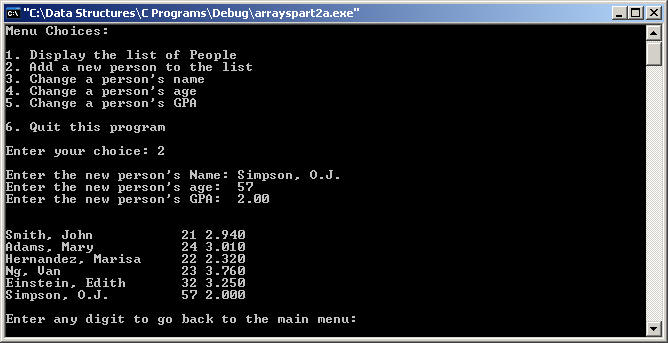
Notice that my program
printed out the new list before returning to the main menu. HOW??
I simply copied and pasted the code I used in my listing routine (above). We
will clean this up later.
There are a few things
you have to be aware of. Cin and cout have some anomolies
associated with them. Using the commands:
cout << "Enter the new person's age:
";
cin >> personage[numberpeople];
Should give you no
problems. However, if you use the command:
cout << "Enter the new person's age:
";
cin >> personage[numberpeople];
You might run into
problems (especially if you enter spaces and other delimiters. So, I will
give you some straight-forward C commands to get the user input and store it
(all you need to do is put this snippet of code in your program):
printf("Enter the
new person's Name: ");
gets(temp);
strcpy(personname[numberpeople], temp);
The only command that
needs a little explanation is strcpy. strcpy is a function
which copies a string to another string. It takes two arguments: (The string
you want to copy to, The string you want to copy from). Actually, it takes:
(The address you want
to copy the string to, The address of the string you want to copy from)
The function is
located in the file string.h, so make sure you include the file at
the top of your file.
Choice 3: Change a
person's name
This is also pretty
easy, but there is something you should do beforehand. You will notice that
choices 3, 4, and 5 require you to first identify the person whose record
the user wants to change. That means you need to get the offset of the array
number, and then replace whatever is in that location with the user input.
Let's take a look at
my output first:
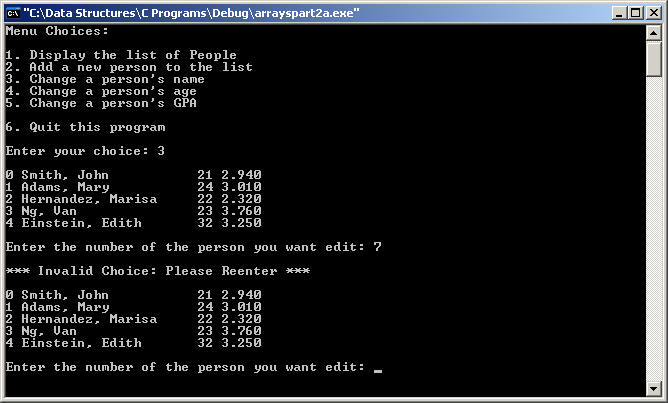
Notice that after menu
option 3 was chosen, I once again displayed the list (A copy and paste job),
but this time with the index/offset number before the person's information.
I then checked to make sure that the user entered a valid number (this uses
the same basic logic that we used to check for a valid menu choice), and
stored it at location personnumber. I didn't let the user get away
with making an invalid choice. If they entered a valid choice, this is what
the output would look like:
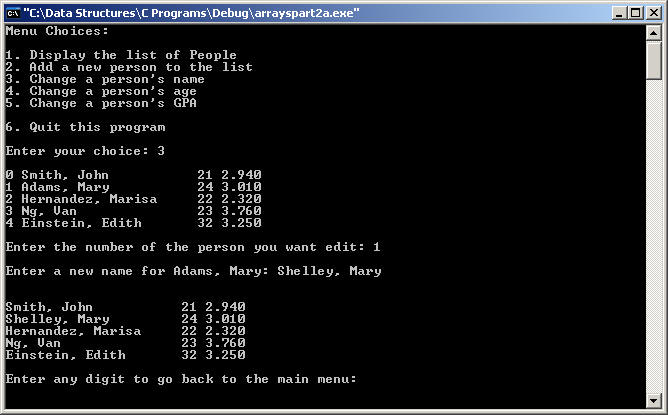
All I did was print
out the name, and get the information. Once again, be careful with cin.
Again, I used the code:
printf("Enter a new
name for %s: , personname[??]);
gets(temp);
strcpy(personname[??], temp);
I think you can figure
out the missing information (and catch the other simple error).
Once again, I printed
out the entire list of people. How?? the same way I did it the other
two times.
Choice 4: Change a
person's age
This is simply a
take-off on changing a person's name (actually much simpler). Once again, I
found out the address where the information is stored (by getting the array
offset), prompting the user, getting the user input, and printing the
information. Here is my output:
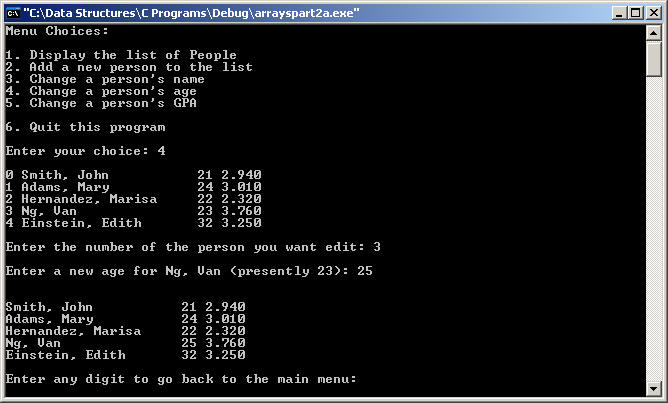
I used the command:
cin >> personage[personnumber]; to get the user input.
Choice 4: Change a
person's GPA
This is almost
identical to choice 3, except that we are getting a real number instead of
an integer. Once again, I found out the address where the information is
stored (by getting the array offset), prompting the user, getting the user
input, and printing the information. Here is my output:
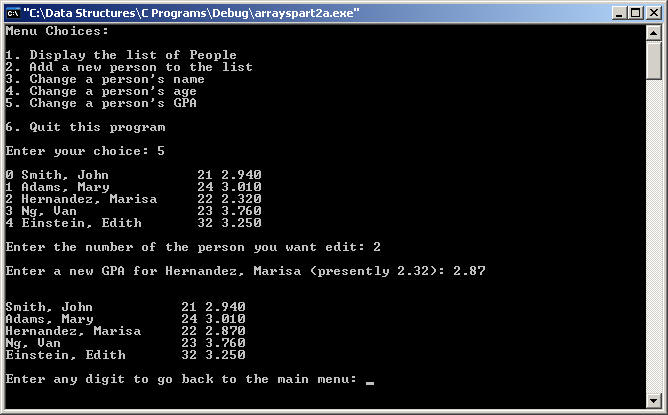
Once again, I used the
cin command.
Get this up and
running, and we will go onto the final part of the assignment.

This page was last updated on
07/06/05